I have added on to this tutorial.
Adjust shipping fee based on quantity in cart.
Adjust shipping fee based on total amount in cart.
Adjust shipping fee based on quantity in cart.
- Setup the WooCommerce -> Settings -> Shipping -> Shipping zones (states/countries),
and the zone name. Then Save changes. - Add a shipping method. Flat rate, Free shipping or Local pickup.
Add two flat rate shipping options.
Tax status None.
Shipping 1 book = $10.
Shipping 2 or more books = $15.
The result:
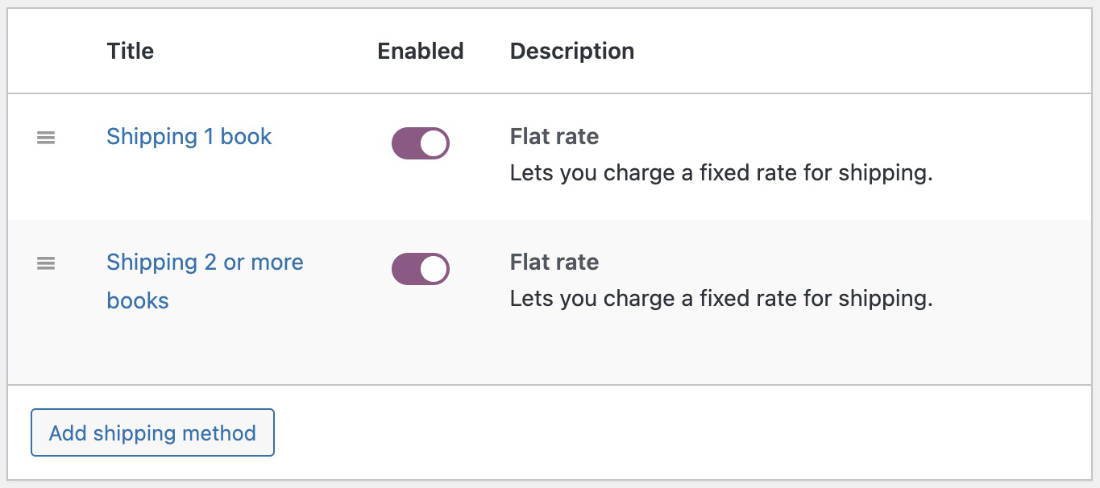
The Cart page Shipping options.
Frontend Cart page: Right clicking Shipping and select through the browser to Inspect (Element). We see the Shipping flate rate is 3 and 4. We need that for the code.
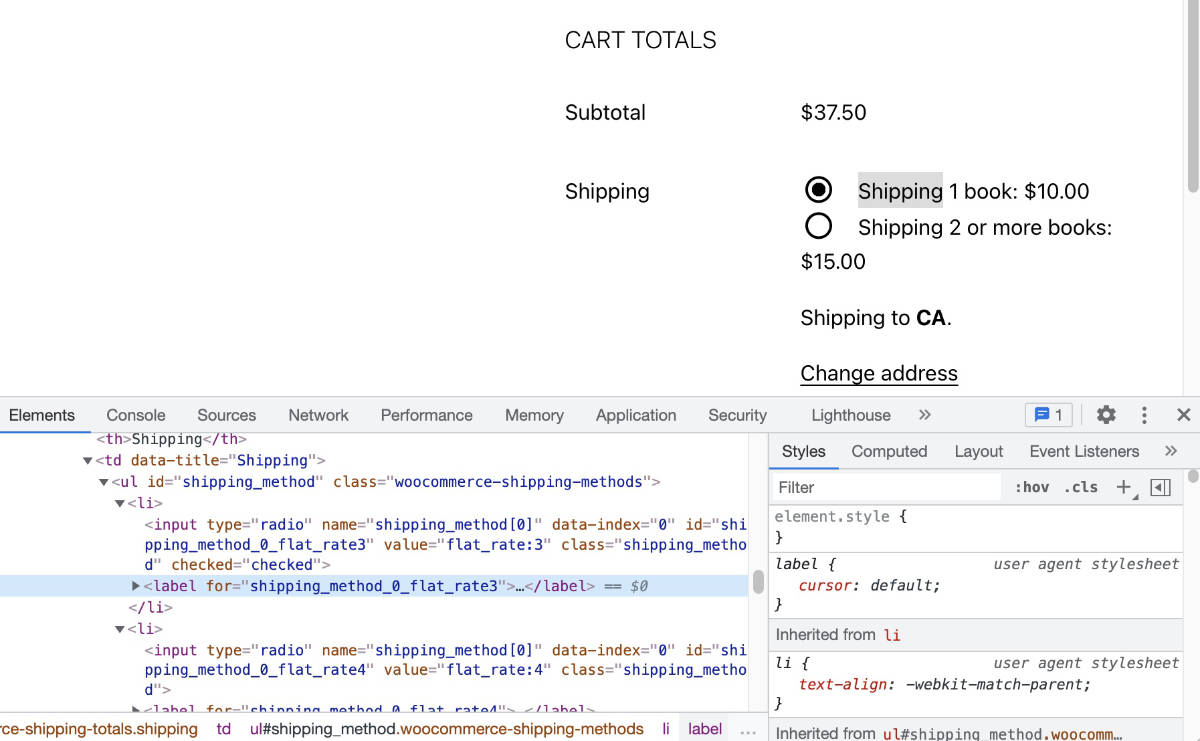
The code used
I based it on the below tutorial but changed amount to cart contents count.
Flat rate 3 = Shipping 1 book.
Flat rate 4 = Shipping 2 or more books.
If threshold = 2 is more than the contents of the cart remove flat rate 4 and use flate rate 3.
/* Code based on: https://www.businessbloomer.com/woocommerce-setup-tiered-shipping-rates-order-amount/ */
add_filter( 'woocommerce_package_rates', 'bbloomer_woocommerce_tiered_shipping', 10, 2 );
function bbloomer_woocommerce_tiered_shipping( $rates, $package ) {
$threshold = 2;
if ( WC()->cart->get_cart_contents_count() < $threshold ) {
// If cart contents 2 or more remove flat rate 3 and use flat rate 4.
unset( $rates['flat_rate:3'] );
} else {
unset( $rates['flat_rate:4'] );
}
return $rates;
}
Adding a third flat rate to the code.
Adjusted flate rates:
Flat rate 3 = Shipping 1 book ($10.00).
Flat rate 4 = Shipping 2 books ($15.00).
Flat rate 5 = Shipping 3 or more books ($18.00).
As before we need to go to the frontend to find the flat rate number for the just added flat rate.
// 3 different flat rates
add_filter( 'woocommerce_package_rates', 'bbloomer_woocommerce_tiered_shipping', 10, 2 );
function bbloomer_woocommerce_tiered_shipping( $rates, $package ) {
$threshold1 = 2;
$threshold2 = 3;
// If cart contents less than 2 unset/remove flat rate 4 and 5.
if ( WC()->cart->get_cart_contents_count() < $threshold1 ) {
unset( $rates['flat_rate:4'], $rates['flat_rate:5'] );
// If cart contents less than 3 unset/remove flat rate 3 and 5.
} elseif ( WC()->cart->get_cart_contents_count() < $threshold2 ){
unset( $rates['flat_rate:3'], $rates['flat_rate:5'] );
// else cart contents 3 or more unset/remove flat rate 3 and 4.
} else {
unset( $rates['flat_rate:3'], $rates['flat_rate:4'] );
}
return $rates;
}
Overview of get_cart_contents codes
get_cart_contents()
get_cart_contents_count()
get_cart_contents_tax()
get_cart_contents_taxes()
get_cart_contents_total()
get_cart_contents_weight()
get_cart_discount_tax_total()
get_cart_discount_total()
get_cart_for_session()
get_cart_from_session()
get_cart_hash()
get_cart_item()
get_cart_item_quantities()
Adjust shipping fee based on total amount in cart.
We can add $threshold of a specific amount. Then unset specific flat rates.
Uses the same flat rates and names as created for the earlier code example.
/* Change shipping based on total cost purchased. */
add_filter( 'woocommerce_package_rates', 'bbloomer_woocommerce_tiered_shipping', 10, 2 );
function bbloomer_woocommerce_tiered_shipping( $rates, $package ) {
// threshold $100 and $200.
$threshold1 = 100;
$threshold2 = 200;
/* unset shipping 2 or more books (flat rate 4) and shipping 3 or more
books (flat rate 5). Uses Shipping 1 book (flat rate 3). */
if ( WC()->cart-> cart_contents_total < $threshold1 ) {
unset( $rates['flat_rate:4'], $rates['flat_rate:5'] );
/* unset shipping 1 book (flat rate 3) and shipping 3 or more
books (flat rate 5). Uses Shipping 2 or more books (flat rate 4). */
} elseif ( WC()->cart-> cart_contents_total < $threshold2 ) {
unset( $rates['flat_rate:3'], $rates['flat_rate:5'] );
/* unset shipping 1 book (flat rate 3) and shipping 2 or more books
(flat rate 4). Uses and shipping 3 or more books (flat rate 5). */
} else {
unset( $rates['flat_rate:3'], $rates['flat_rate:4'] );
}
return $rates;
}
Resources:
https://woocommerce.com/documentation/plugins/woocommerce/getting-started/shipping/
https://woocommerce.com/document/flat-rate-shipping/
https://woocommerce.com/document/product-shipping-classes/
https://woocommerce.github.io/code-reference/classes/WC-Cart.html#method_get_cart_contents
https://wpastra.com/woocommerce-shipping/
https://www.businessbloomer.com/woocommerce-flat-rate-by-weight/
This tutorial was originally written 11 September 2018.