Creating parallax effects when scrolling with WordPress
A static jpg showing 4 product images. These can be animated.
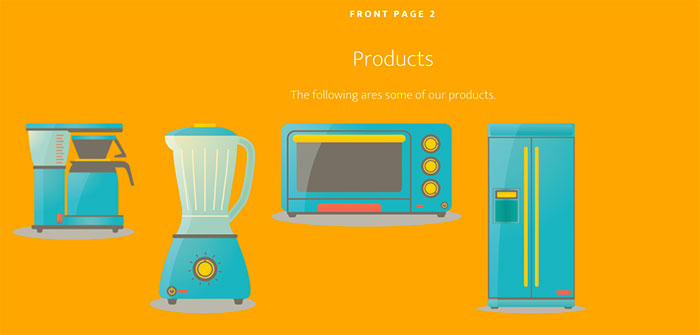
I am using two files. One to create the main animation effects (Waypoints) and a CSS file (Animate) to style the animations.
Download: https://imakewebthings.com/waypoints/ and https://github.com/daneden/animate.css.
Copy/move the lib/jquery.waypoints.min.js file to a child theme js folder (create a js folder if one does not exist). Copy/move the animate.css file to the child theme root folder (or to a CSS folder).
waypoints-init.js
Create a waypoints-init.js file and add it inside the js folder of your child theme.
I am using Altitude Pro from Genesis as an example on a one page theme.
It consists of various front page sections. As in #front-page-1, 2, 3, 4 etc.
Add widgets with headings h1, some text using p and images.
Add the following code:
// Resources:
// https://github.com/daneden/animate.css
// https://spin.atomicobject.com/2015/05/31/scroll-anmiation-css-waypoints/
jQuery(function($) {
$('#front-page-1').waypoint(function(direction) {
if (direction == 'down') {
$('#front-page-1 h1').toggleClass( 'animated fadeInUp' );
$('#front-page-1 img').addClass('animated fadeInLeft');
$('#front-page-1 p').toggleClass('animated fadeInUp');
}
if (direction == 'up') {
$('#front-page-1 h1').toggleClass( 'animated fadeOut' );
}
},
{
offset: '80%',
});
$('#front-page-2 img').css('opacity', 0);
$('#front-page-2 h1').css('opacity', 0);
$('#front-page-2').waypoint(function() {
$('#front-page-2 h1').toggleClass( 'animated fadeInUp' );
// $('#front-page-2 img').toggleClass('animated fadeInUpBig');
// 4 products being animated.
$('#front-page-2 .wp-image-81').toggleClass('animated fadeInLeft');
$('#front-page-2 .wp-image-77').toggleClass('animated fadeInUp');
$('#front-page-2 .wp-image-78').toggleClass('animated rotateInDownLeft');
$('#front-page-2 .wp-image-79').toggleClass('animated fadeInRight');
},
{
offset: '30%',
});
$('#front-page-3 h1').css('opacity', 0);
$('#front-page-3').waypoint(function() {
$('#front-page-3 h1').toggleClass( 'animated fadeIn' );
$('#front-page-3 img').toggleClass( 'animated fadeInLeftBig' );
},
{
offset: '40%',
});
$('#front-page-4').waypoint(function() {
$('#front-page-4 h1').toggleClass( 'animated fadeIn' );
$('#front-page-4 p').toggleClass( 'animated fadeInLeftBig' );
$('#front-page-4 img').toggleClass( 'animated fadeIn' );
},
{
offset: '80%',
});
$('#front-page-7').waypoint(function() {
$('#front-page-7 p').addClass( 'animated fadeInUpBig' );
},
{
offset: '60%',
});
});
Functions.php file inside the child theme.
Add the following code:
//* Enqueue scripts and styles
add_action( 'wp_enqueue_scripts', 'themename_enqueue_scripts_styles' );
function themename_enqueue_scripts_styles() {
// I added the following code
//* Enqueue Parallax on non handhelds i.e., desktops, laptops etc. and not on tablets and mobiles
// Source: https://daneden.github.io/animate.css/
wp_enqueue_style( 'animate', get_stylesheet_directory_uri() . '/animate.css' );
wp_enqueue_script( 'waypoints', get_stylesheet_directory_uri() . '/js/jquery.waypoints.min.js', array( 'jquery' ), '1.0.0' );
wp_enqueue_script( 'waypoints-init', get_stylesheet_directory_uri() .'/js/waypoints-init.js' , array( 'jquery', 'waypoints' ), '1.0.0' );
}
An overview.
animate.css is in the root of the child theme folder.
jquery.waypoints.min.js and waypoints-init.js are in the js folder.
Exploration
In waypoints-init.js I added the following code.
$('#front-page-2').waypoint(function() {
$('#front-page-2 h1').toggleClass( 'animated fadeInUp' );
// $('#front-page-2 img').toggleClass('animated fadeInUpBig');
// 4 products being animated.
$('#front-page-2 .wp-image-81').toggleClass('animated fadeInLeft');
$('#front-page-2 .wp-image-77').toggleClass('animated fadeInUp');
$('#front-page-2 .wp-image-78').toggleClass('animated-3 fadeInDown');
$('#front-page-2 .wp-image-79').toggleClass('animated-4 fadeInRight');
},
{
offset: '30%',
});
In animate.css I added the following
/* 3 image */
.animated-3{
-webkit-animation-fill-mode:both;
-moz-animation-fill-mode:both;
-ms-animation-fill-mode:both;
animation-fill-mode:both;
-webkit-animation-duration:2.5s;
-moz-animation-duration:2.5s;
-ms-animation-duration:2.5s;
animation-duration:2.5s;
}
/* 4 image */
.animated-4{
-webkit-animation-fill-mode:both;
-moz-animation-fill-mode:both;
-ms-animation-fill-mode:both;
animation-fill-mode:both;
-webkit-animation-duration:4s;
-moz-animation-duration:4s;
-ms-animation-duration:4s;
animation-duration:4s;
}
By changing the duration I am also delaying the animation a little between some of the images.
Resources:
https://coderwall.com/p/nuzcua/how-i-delayed-timed-animate-css-animations
https://medium.com/@samburgers/full-screen-sticky-css-5d9d5d77afec#.dfqb8o75m