How to modify the top admin toolbar menu in WordPress.
The following approach uses code to be added to a custom plugin, a core snippet plugin or in a child theme funtions.php file. One can also use CSS to hide various links instead of this approach.
WordPress Codex resources:
https://wordpress.org/support/article/toolbar/
https://developer.wordpress.org/reference/classes/wp_admin_bar/add_node/
WordPress Core trac ticket:
https://core.trac.wordpress.org/ticket/32678
Earlier WordPress core explorations.
https://github.com/helen/wp-toolbar-experiments
The mission was to make the top admin toolbar even more useful.
Finding the CSS ID of a node
Finding the node we use Inspect (element). On the frontend of a site you are logged into, right click the browser in the top admin area of the WordPress site and select Inspect. This will open the HTML and CSS sections of the site.
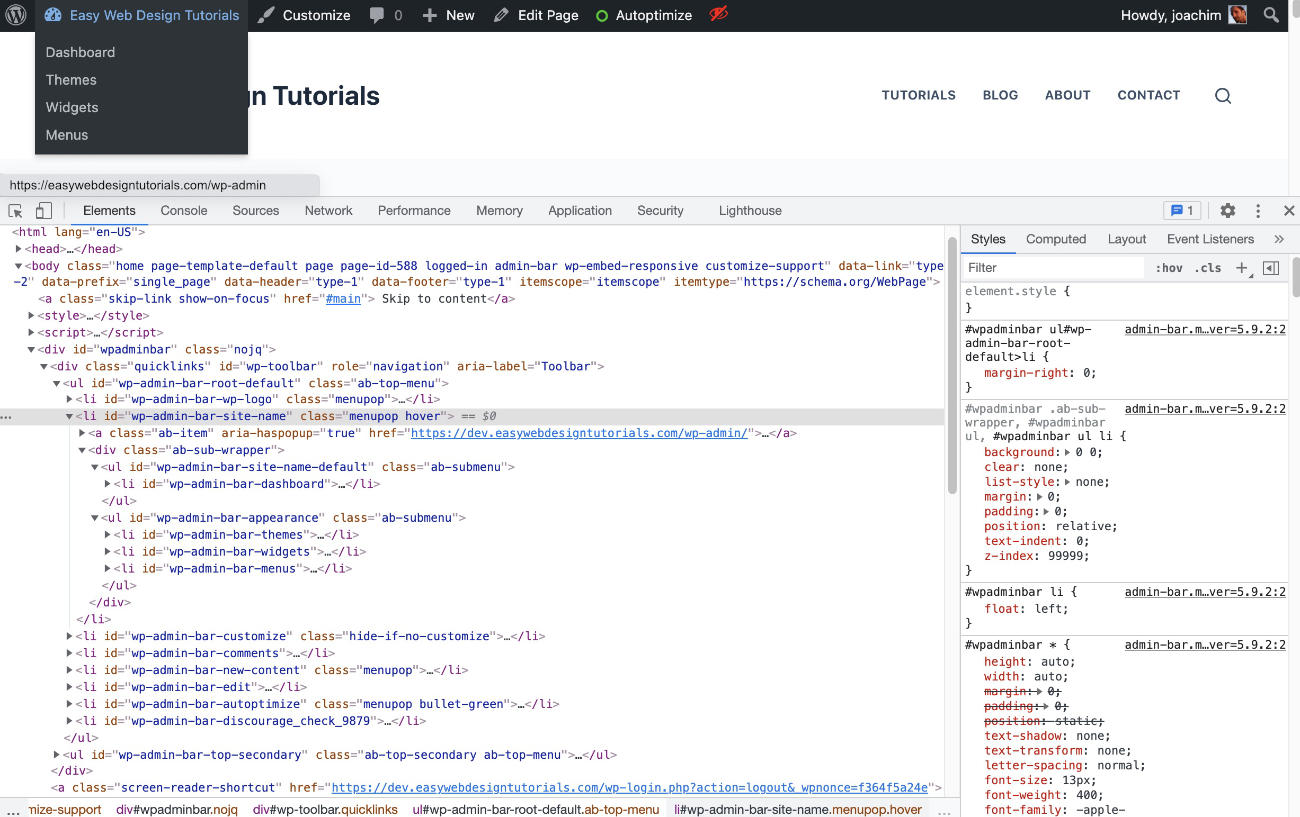
Admin bar CSS ID link hierarchy.
To find the node remove: wp-admin-bar from the CSS ID.
wpadminbar
- wp-toolbar
-- wp-admin-bar-root-default
--- wp-admin-bar-wp-logo
---- (CSS class) ab-sub-wrapper
----- wp-admin-bar-wp-logo-default
------ wp-admin-bar-about
----- wp-admin-bar-wp-logo-external
------ wp-admin-bar-wporg
------ wp-admin-bar-documentation
------ wp-admin-bar-support-forums
------ wp-admin-bar-feedback
--- wp-admin-bar-site-name
---- (CSS class) ab-sub-wrapper
----- wp-admin-bar-site-name-default
----- wp-admin-bar-appearance
------ wp-admin-bar-themes
------ wp-admin-bar-widgets
------ wp-admin-bar-menus
--- wp-admin-bar-customize
--- wp-admin-bar-comments
--- wp-admin-bar-new-content
---- (CSS class) ab-sub-wrapper
----- wp-admin-bar-new-content-default
------ wp-admin-bar-new-post
------ wp-admin-bar-new-media
------ wp-admin-bar-new-page
------ wp-admin-bar-new-tutorials
------ wp-admin-bar-new-user
--- wp-admin-bar-edit
--- wp-admin-bar-autoptimize (Added by Autoptimize plugin)
--- wp-admin-bar-discourage_check_9879 (Added by Discourage search engines plugin)
-- wp-admin-bar-top-secondary
--- wp-admin-bar-search
--- wp-admin-bar-my-account
---- (CSS class) ab-sub-wrapper
----- wp-admin-bar-user-actions
------ wp-admin-bar-user-info
------ wp-admin-bar-edit-profile
------ wp-admin-bar-logout
Adding a sub menu link to the site name.
Let’s add the Media Library link to the site name submenu.
/* Add a media library sub menu link to the site name menu.*/
add_action( 'admin_bar_menu', 'add_link_to_admin_bar',999 );
function add_link_to_admin_bar($wp_admin_bar) {
// adds a child node to site name parent node
$wp_admin_bar->add_node( array(
'parent' => 'site-name',
'id' => 'media-library',
'title' => 'Media Library',
'href' => esc_url( admin_url( 'upload.php' ) ),
'meta' => false
));
}
Adding two sub menu links to the site name.
/* Add a media library and plugins sub menu link to the site name menu.*/
add_action( 'admin_bar_menu', 'add_link_to_admin_bar',999 );
function add_link_to_admin_bar($wp_admin_bar) {
// adds a child node to site name parent node
$wp_admin_bar->add_node( array(
'parent' => 'site-name',
'id' => 'media-library',
'title' => 'Media Library',
'href' => esc_url( admin_url( 'upload.php' ) ),
'meta' => false
));
// adds the child node plugins to site name parent node
$wp_admin_bar->add_node( array(
'parent' => 'site-name',
'id' => 'plugins',
'title' => 'Plugins',
'href' => esc_url( admin_url( 'upload.php' ) ),
'meta' => false
));
}
The Result of adding Media Library and Plugins links to the Site name submenu.
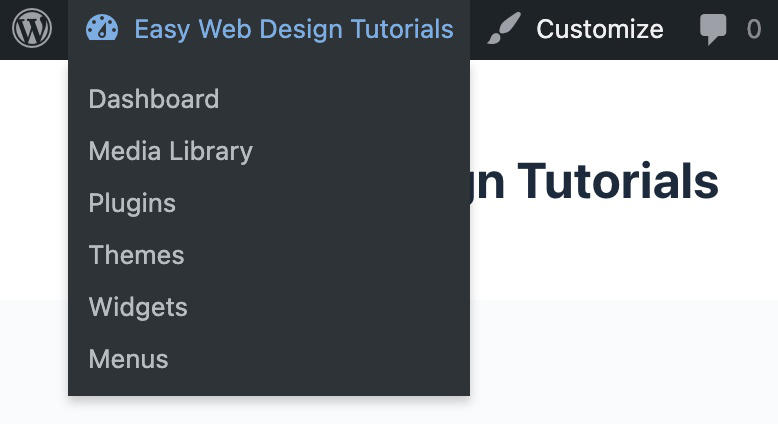
Adding a top level link and two submenu links.
/* Add a top level link I named Custom Made having it link to an external web site and two sub menu links which go to the media library and plugins. */
add_action( 'admin_bar_menu', 'add_nodes_to_admin_bar',999 );
function add_nodes_to_admin_bar($wp_admin_bar) {
// adds a top level parent node I called Custom Made
$wp_admin_bar->add_node( array(
'id' => 'custom',
'title' => 'Custom Made',
'href' => 'http://easywebdesigntutorials.com/',
));
// adds the link media library to Custom Made
$wp_admin_bar->add_node( array(
'parent' => 'custom',
'id' => 'media-lib', // If adding the same link twice in the admin bar the second id needs a unique name.
'title' => 'Media Library',
'href' => esc_url( admin_url( 'upload.php' ) ),
'meta' => false
));
// adds the child node plugins to Custom Made
$wp_admin_bar->add_node( array(
'parent' => 'custom',
'id' => 'plug', // If adding the same link twice in the admin bar the second id needs a unique name.
'title' => 'Plugins',
'href' => esc_url( admin_url( 'plugins.php' ) ),
'meta' => false
));
}
The result of adding a new top level link and two submenu items.
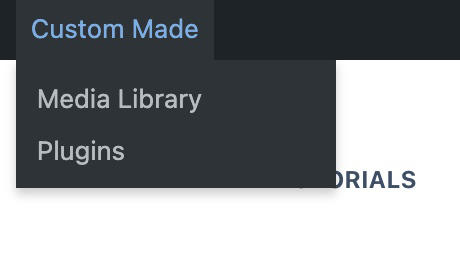
Remove the top level customize and comments links.
/* Remove the top level customize and comments link. */
add_action( 'admin_bar_menu', 'remove_customize',999 );
function remove_customize ($wp_admin_bar) {
// Remove customize link from the top level admin menu bar.
$wp_admin_bar->remove_node( 'customize' );
// Remove comments link from the top level admin menu bar.
$wp_admin_bar->remove_node( 'comments' );
}
The result of removing customize and comments links from the top level admin bar.

Adjust top admin bar only on frontend and not on backend.
The following code will only remove comments from the frontend and not the backend.
/* Remove the top level comments links only on frontend. */
add_action( 'admin_bar_menu', 'remove_comments',999 );
if ( ! is_admin() ) { //If not admin (backend) then continue.
function remove_comments ($wp_admin_bar) {
// Remove comments link from the top level admin menu bar.
$wp_admin_bar->remove_node( 'comments' );
}
}
Remove the top admin toolbar.
It becomes hidden from view.
/* Remove the admin toolbar. */
add_filter('show_admin_bar', '__return_false');
A few Admin Toolbar plugins:
Admin Menu Editor plugin
Admin Toolbar Menus
Auto Hide Admin Toolbar
Admin Bar plus
Other helpful resources that also have additional customizations of the admin toolbar.
https://heera.it/customize-admin-menu-bar-in-wordpress
https://stackoverflow.com/questions/21614230/wordpress-admin-bar-not-displaying-both-custom-drop-down-menus
https://digwp.com/2011/04/admin-bar-tricks/
A special thank you to Drew for helping me with the code.
Admin Toolbar correct coding (to be used in the codex).
This tutorial was originally written 14 May 2015.