Here is an example of a single / individual product page in WooCommerce.
Using the default theme Twenty Twenty Two.
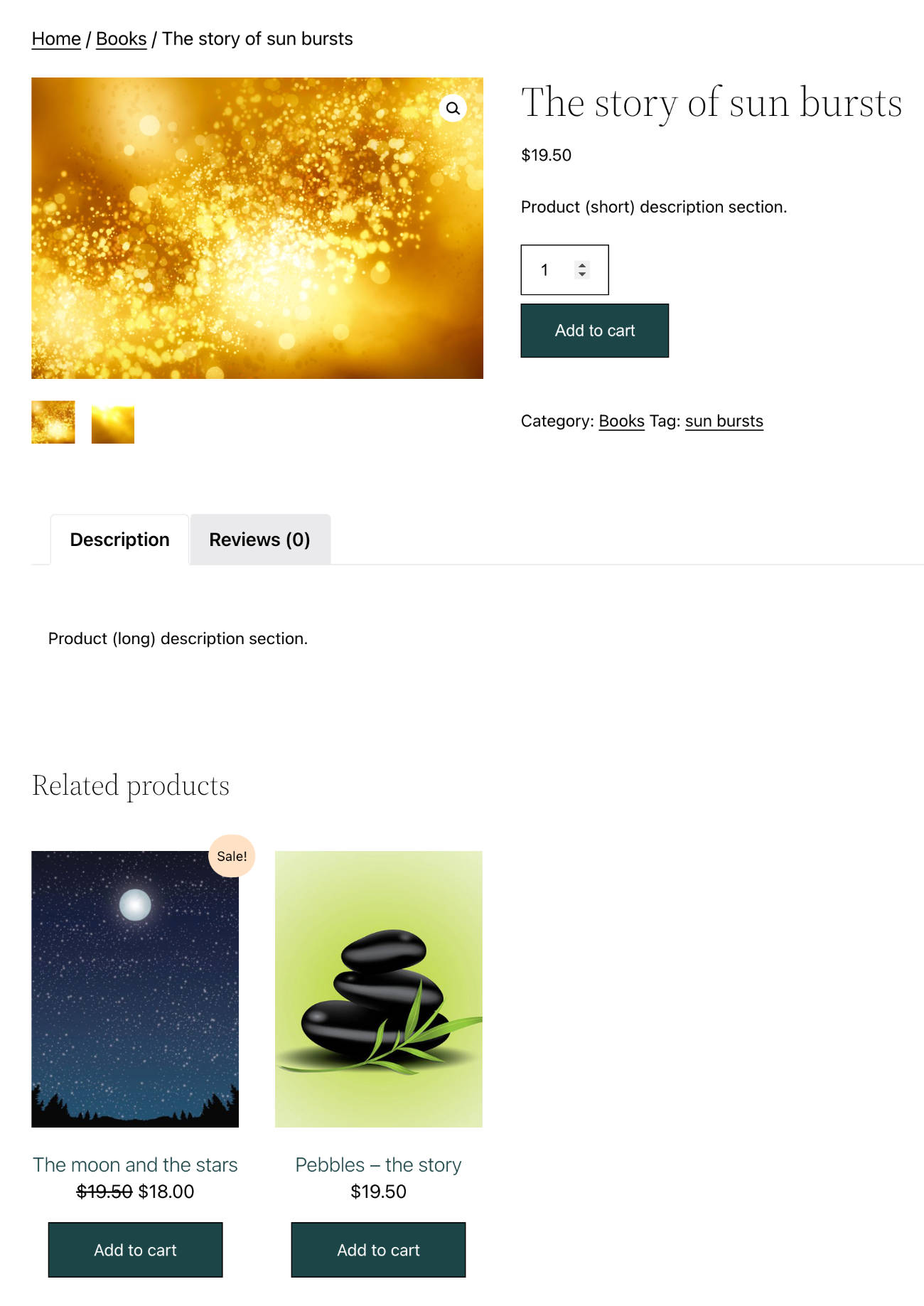
There are different ways to add code. Through a code snippet plugin, through the customizer, in a custom plugin or through a child theme functions.php file and style.css file.
The product image
We see the product image with zoom features. The following code examples removes the zoom, lightbox and slider effects.
/* Disable the magnification zoom, lightbox and slider. Add the code to the functions file. https://github.com/woocommerce/woocommerce/issues/18131#issuecomment-392242855 */
add_action( 'template_redirect', function() {
remove_theme_support( 'wc-product-gallery-zoom' );
remove_theme_support( 'wc-product-gallery-lightbox' );
remove_theme_support( 'wc-product-gallery-slider' );
}, 100 );
Make the product image non clickable with CSS.
/* Make the product image non clickable. */
.woocommerce div.product div.woocommerce-product-gallery figure.woocommerce-product-gallery__wrapper {
margin: 0;
pointer-events: none !important;
}
Using a video instead of the product image.
/* Switching the product image with a video.
https://www.businessbloomer.com/woocommerce-show-video-instead-featured-image/ */
function bbloomer_show_video_not_image() {
// Do this for product ID = 11 only
if ( is_single( '11' ) ) {
remove_action( 'woocommerce_before_single_product_summary', 'woocommerce_show_product_images', 20 );
remove_action( 'woocommerce_product_thumbnails', 'woocommerce_show_product_thumbnails', 20 );
add_action( 'woocommerce_before_single_product_summary', 'bbloomer_show_product_video', 20 );
}
}
function bbloomer_show_product_video() {
echo '<div class="woocommerce-product-gallery">';
// get video embed HTML from YouTube
echo '<iframe width="480" height="315" src="https://www.youtube.com/embed/JHN7viKRxbQ?rel=0&showinfo=0" frameborder="0" allowfullscreen></iframe>';
echo '</div>';
}
CSS video adjustments.
/* CSS adjustment for adding a video instead of product image. */
.woocommerce div.product div.woocommerce-product-gallery {
position: relative;
width: 500px;
float: left;
}
The result of using a video instead of an image in the single product page:

Make the product image go full width.
CSS only adjustments.
/* Make the product image go full width. Pushes the other information below the image.
https://www.businessbloomer.com/woocommerce-full-width-featured-image-single-product-page/ */
.woocommerce #content div.product div.images, .woocommerce div.product div.images, .woocommerce-page #content div.product div.images, .woocommerce-page div.product div.images, .woocommerce #content div.product div.summary, .woocommerce div.product div.summary, .woocommerce-page #content div.product div.summary, .woocommerce-page div.product div.summary {
float: none;
width: 100%;
max-width: unset;
clear: both;
}
.woocommerce-product-gallery img {
width: 100%;
}
Product information to the right of the image.
To the right of the product image we have the title, price, short description, quantity, Add to cart button, category and tags. One can rename, reorder or remove any of these sections.
Moving the title and product description
Each section is has a priority number associated with it to tell WooCommerce where these should be located in relation to each other. These are called hooks.
WooCommerce Single Product Page [Visual Hook Guide]
Businessbloomer woocommerce visual hook guide single product page.
Top:
woocommerce_before_single_product
woocommere_before_single_product_summary
Below product image:
woocommerce_product_thumbnails (may not work)
Right product area:
woocommerce_single_product_summary
woocommerce_before_add_to_cart_form
woocommerce_before_variations_form
woocommerce_before_add_to_cart_button
woocomerce_before_single_variation
woocoomerce_single_variation
woocommerce_before_add_to_cart_quantity
woocommerce_after_add_to_cart_quantity
woocommerce_after_single_variation
woocommerce_after_add_to_cart_button
woocommerce_after_variations_form
woocommerce_after_add_to_cart_form
woocommerce_product_meta_start
woocommerce_product_meta_end
woocommerce_share
After product area:
woocommerce_after_single_product_summary
woocommerce_after_single_product
woocommerce_template_single_title – 5
woocommerce_template_single_price – 10
woocommerce_template_single_excerpt – 20
woocommerce_template_single_add_to_cart – 30
woocommerce_template_single_meta – 40
woocommerce_template_single_sharing – 50
To change the priority, you need to remove the hook and add it again with a new number. This will change where a section is located.
Some examples.
/* Move the product price from above the product short description section to below the short description. */
remove_action('woocommerce_single_product_summary', 'woocommerce_template_single_price', 10);
add_action('woocommerce_single_product_summary', 'woocommerce_template_single_price', 20);
/* Move the product title to above the product image. The code will remove the title from the woocommerce_single_product_summary section and place it into the woocommerce_before_single_product_summary section. */
remove_action('woocommerce_single_product_summary', 'woocommerce_template_single_title', 5);
add_action('woocommerce_before_single_product_summary', 'woocommerce_template_single_title', 5);
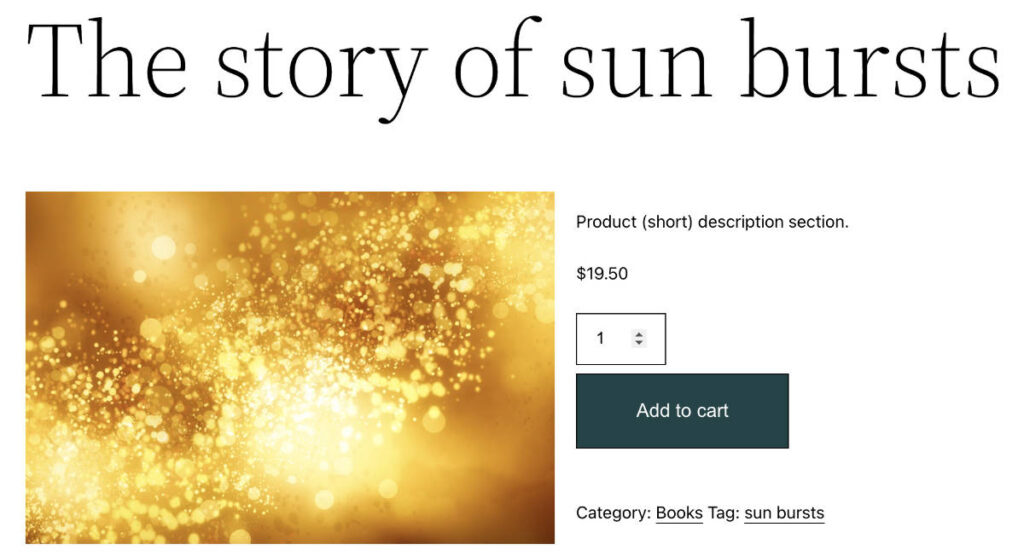
The following will hide the meta section. Categories, Tags and SKU number.
/* Remove the meta section. Hide the SKU number, category and tags.
https://www.businessbloomer.com/woocommerce-hide-category-tag-single-product-page/ */
remove_action( 'woocommerce_single_product_summary', 'woocommerce_template_single_meta', 40 );
/* Remove only the SKU number from the frontend.
https://www.businessbloomer.com/woocommerce-hide-sku-front-end/ */
add_filter( 'wc_product_sku_enabled', 'bbloomer_remove_product_page_sku' );
function bbloomer_remove_product_page_sku( $enabled ) {
if ( ! is_admin() && is_product() ) {
return false;
}
return $enabled;
}
/* CSS: hide the product meta */
.single-product div.product .product_meta {
display: none;
}
/* CSS: Remove "In Stock" label and number. */
.woocommerce div.product .stock {
display: none;
}
Add custom text above the Add to Cart selection and button.
/* Add custom text above add to cart selection and button.
https://www.businessbloomer.com/woocommerce-add-text-add-cart-single-product-page/ */
add_action( 'woocommerce_single_product_summary', 'bbloomer_show_return_policy', 20 );
function bbloomer_show_return_policy() {
echo '<p class="custom-text">Custom text above add to cart number selection and button.</p>';
}
Adding text and link.
/* A variation of the above which also includes adding a link to the text. https://quadlayers.com/edit-woocommerce-product-page-programmatically/ */
add_action( 'woocommerce_single_product_summary', 'woo_text_link', 20 );
function woo_text_link() {
echo( '<p>Some text here. <a href="#" class="custom-text">A custom link!</a></p>');
}
Add the CSS to the class “custom-text”.
/* CSS class added to the above code. Can be styled in the style.css file. */
.custom-text {
font-style: italic;
color: red;
}
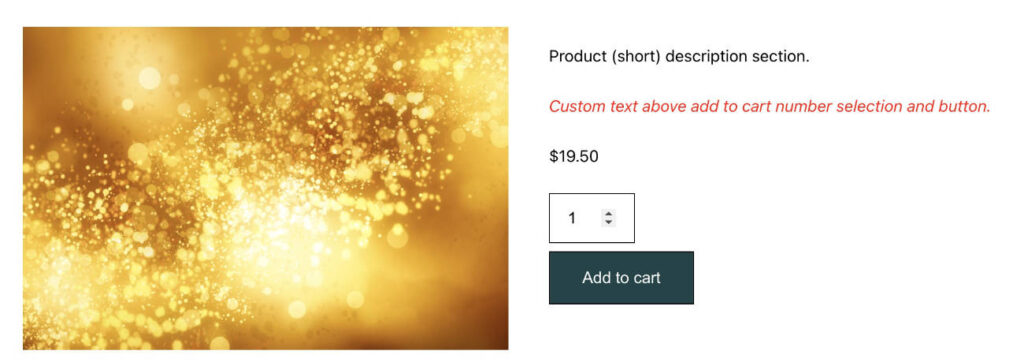
Adjust “Add to cart” text.
/* Change "Add to cart" text. https://quadlayers.com/customize-add-to-cart-button-woocommerce/ */
add_filter('woocommerce_product_single_add_to_cart_text', 'custom_add_to_cart_text');
function custom_add_to_cart_text() {
return __('Buy Now', 'woocommerce');
}
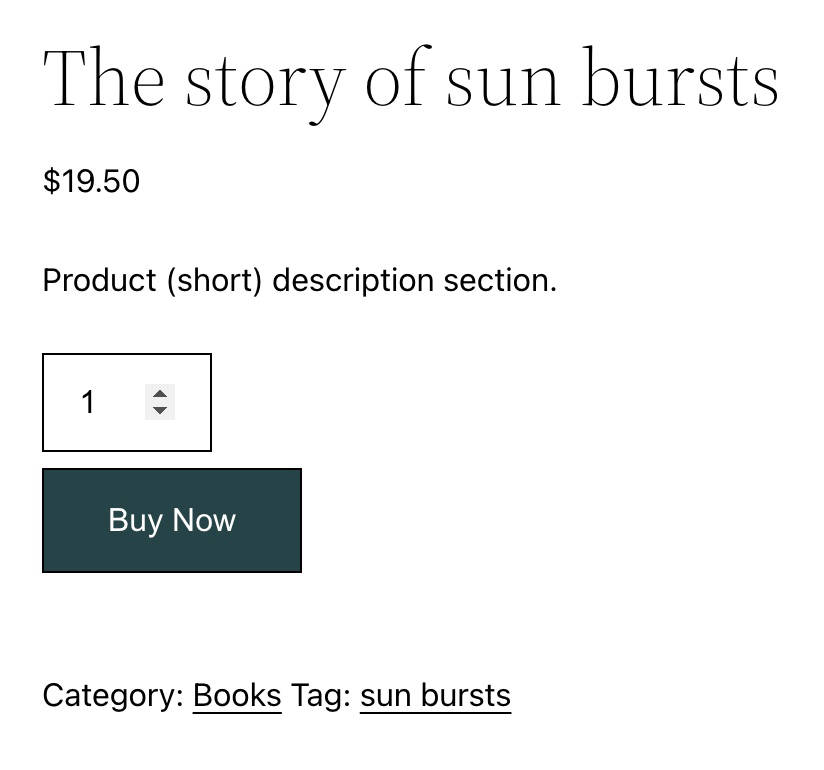
Change “Add to cart” button text based on product category.
/* Change "Add to cart" button text based on category. https://www.businessbloomer.com/woocommerce-edit-add-to-cart-text-by-product-category/ */
add_filter( 'woocommerce_product_single_add_to_cart_text', 'bbloomer_single_custom_cart_button_text' );
function bbloomer_single_custom_cart_button_text() {
global $product;
if ( has_term( 'Books', 'product_cat', $product->ID ) ) {
return 'Add a book';
} elseif ( has_term( 'Movies', 'product_cat', $product->ID ) ) {
return 'Add a movie';
} else {
return 'Add to cart';
}
}
Tabs section – below product image.
Here are various modifications that can be done with the tabs seen below the product image area in WooCommerce.
Rename tab – “Description”.
To rename “Additional Information” or “Reviews” tabs adjust the add filter name from description to additional_information or reviews.
/* Rename tab - description. https://www.businessbloomer.com/woocommerce-edit-product-tabs-labels/ */
add_filter( 'woocommerce_product_description_tab_title', 'bbloomer_rename_description_product_tab_label' );
function bbloomer_rename_description_product_tab_label() {
return 'Rename the description tab';
}
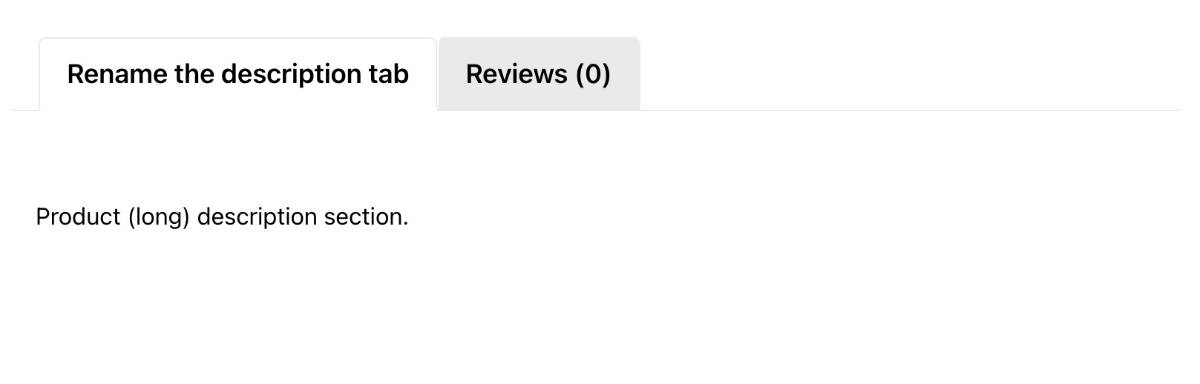
Remove the word “Description” from inside the Description tab.
/* Remove the word "description" h2 heading inside the Description tab. https://www.businessbloomer.com/woocommerce-remove-description-heading-tabs/ */
add_filter( 'woocommerce_product_description_heading', '__return_null' );
Hide “Description”, “Additional Information” and “Reviews” tabs.
/* Hide "Description", "Additional Information" and "Reviews" tabs https://www.businessbloomer.com/woocommerce-remove-additional-info-tab-products/ */
add_filter( 'woocommerce_product_tabs', 'bbloomer_remove_tab', 9999 );
function bbloomer_remove_tab( $tabs ) {
unset( $tabs['description'] );
unset( $tabs['additional_information'] );
unset( $tabs['reviews'] );
return $tabs;
}
Related Products section
Move “Related products” to become a new tab.
/* Move "Related products" from below the tabs to become a new tab. https://www.businessbloomer.com/woocommerce-related-products-tab/ */
// 1 - Remove related products from their original position.
remove_action( 'woocommerce_after_single_product_summary', 'woocommerce_output_related_products', 20);
// 2 - add a new tab.
add_filter( 'woocommerce_product_tabs', 'woo_new_product_tab' );
function woo_new_product_tab( $tabs ) {
$tabs['related_products'] = array(
'title' => __( 'Related products', 'woocommerce' ),
'priority' => 50,
'callback' => 'woo_new_product_tab_content'
);
return $tabs;
}
// 3 - place the related products inside.
function woo_new_product_tab_content() {
woocommerce_output_related_products();
}
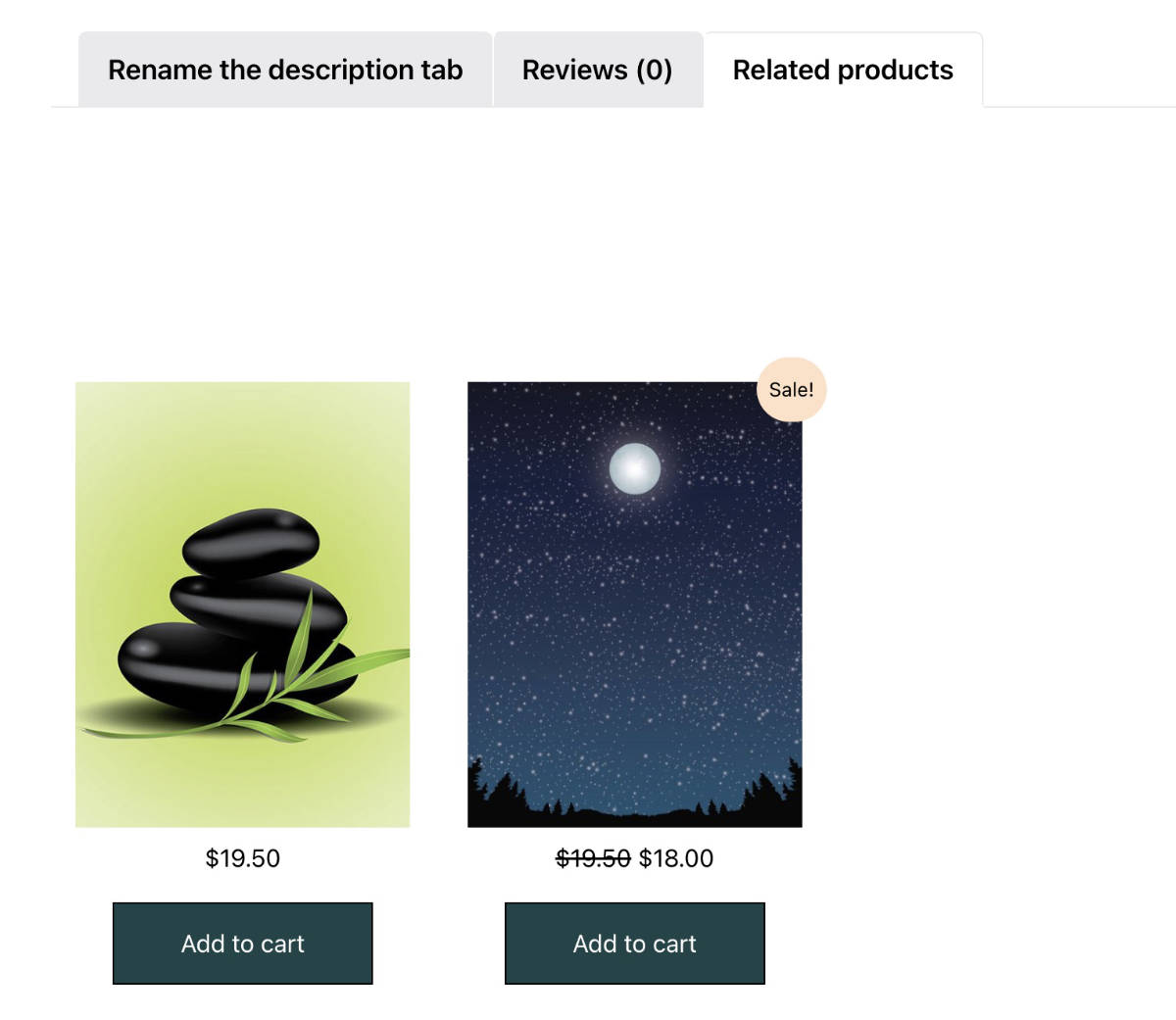
Change number of related product output.
/* Change number of related products output: https://gist.github.com/eddhurst/d148b8a93c16acdeb8fd3865e5d8eb9f */
add_filter( 'woocommerce_output_related_products_args', 'woo_related_products_limit', 20 );
function woo_related_products_limit() {
global $product;
$args['posts_per_page'] = 4; // Only show 4 related products.
$args['columns'] = 4; // arranged in 2 columns.
return $args;
}
Hide “Related products”.
/* Hide "Related products" https://www.businessbloomer.com/remove-woocommerce-related-products/ */
remove_action( 'woocommerce_after_single_product_summary', 'woocommerce_output_related_products', 20 );
Rename “Related products” text.
/* Rename "Related products" :
https://stackoverflow.com/questions/45688753/rename-related-products-title-in-woocommerce-3 */
add_filter('woocommerce_product_related_products_heading',function(){
return 'My Custom nice related title';
});
Direct code snippet translation.
Related products is directly translated into Norwegian “You might perhaps also like….”.
/* -------- Related posts --------- */
// Change WooCommerce "Related products" text - https://themeskills.com/change-related-products-text-woocommerce/
add_filter('gettext', 'change_rp_text', 10, 3);
add_filter('ngettext', 'change_rp_text', 10, 3);
function change_rp_text($translated, $text, $domain)
{
if ($text === 'Related products' && $domain === 'woocommerce') {
$translated = esc_html__('Du liker kanskje også…', $domain);
}
return $translated;
}
Additional resources
https://www.businessbloomer.com/woocommerce-visual-hook-guide-single-product-page/
https://wisdmlabs.com/blog/reorder-content-woocommerce-product-page/
https://www.businessbloomer.com/woocommerce-checkbox-to-disable-related-products-conditionally/
https://quadlayers.com/edit-woocommerce-product-page-programmatically/
https://www.businessbloomer.com/woocommerce-add-content-below-the-single-product-page-images/