Part 1: Modifications of the current fields seen in the checkout page.
We are now at part 2: Add custom fields to the Checkout page.
Here is the default Checkout page seen in the theme Twenty Twenty Two.
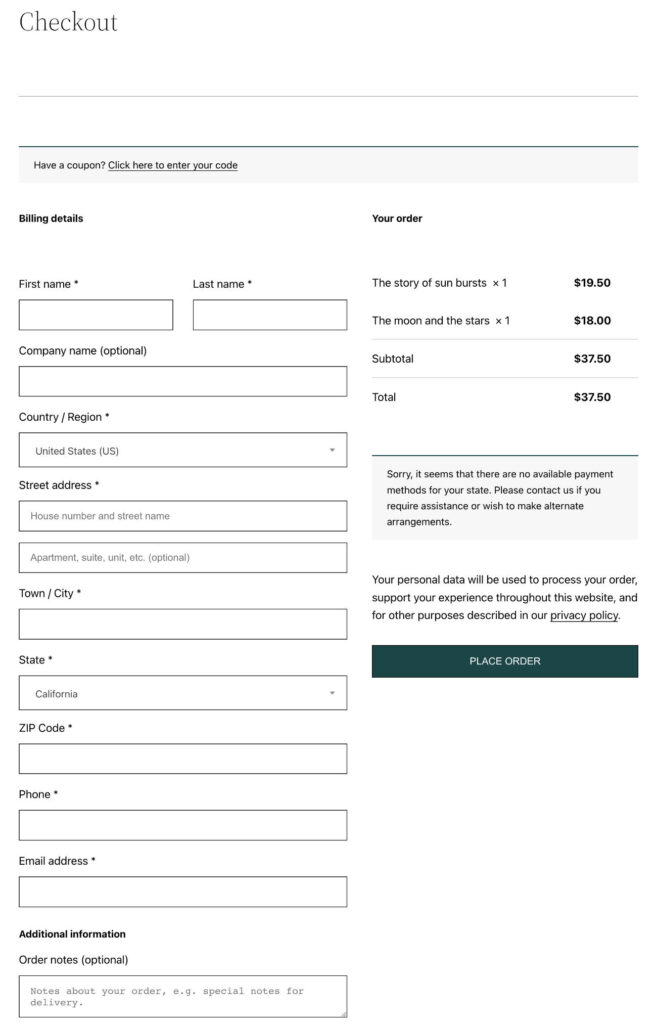
Custom field types in WooCommerce
country, state, text, textarea, select (dropdown), radio, checkbox, password, datetime, datetime-local, date, month, time, week, number, email, url and tel.
Options one can add inside the array.
/* https://pluginrepublic.com/woocommerce-custom-fields/ and https://iconicwp.com/blog/the-ultimate-guide-to-adding-custom-woocommerce-user-account-fields/ */
$args = array(
'type' => 'text',
'label' => '',
'description' => '',
'placeholder' => '',
'maxlength' => false,
'required' => false,
'autocomplete' => false,
'id' => $key,
'class' => array(),
'label_class' => array(),
'input_class' => array(),
'return' => false,
'options' => array(),
'custom_attributes' => array(),
'validate' => array(),
'default' => '',
'autofocus' => '',
'priority' => '',
);
Adding the custom fields to the Checkout page.
I have added text, text area, date and checkboxes as custom fields. Labels and for instance billing_namehere can be adjusted. Explore and use the resources I linked to in addition to the tutorial that I wrote. Here is the code I used.
/* Adding custom fields: checkbox, date, drop down, text and text area. */
add_filter( 'woocommerce_checkout_fields', 'custom_fields_checkout' );
function custom_fields_checkout( $fields ) {
// Added below the billing area.
$fields['billing']['billing_age'] = array(
'label' => 'I am 18 or over.',
'type' => 'checkbox',
//'default' => 1, //This will pre-select the checkbox
'required' => true,
'class' => array( 'form-row-wide' ),
'priority' => 25,
);
$fields['billing']['billing_date'] = array(
'label' => 'Service Date',
'type' => 'date',
'required' => true,
'class' => array( 'form-row-wide' ),
'priority' => 26,
);
$fields['billing']['billing_dropdown'] = array(
'label' => 'Drop Down',
'required' => true,
'class' => array( 'form-row-wide' ),
'priority' => 27,
'type' => 'select',
'options' => array( // options for <select> or <input type="radio" />
'' => 'Please select Service',
// empty values means that field is not selected
// 'value'=>'Name'
'Service option 1' => 'Service option 1',
'Service option 2' => 'Service option 2',
'Service option 3' => 'Service option 3'
)
);
$fields['billing']['billing_text'] = array(
'label' => 'Special needs',
'type' => 'text',
'required' => true,
'class' => array( 'form-row-wide' ),
'priority' => 28,
);
$fields['billing']['billing_textarea'] = array(
'label' => 'Comments about delivery',
'type' => 'textarea',
'required' => true,
'class' => array( 'form-row-wide' ),
'priority' => 29,
);
// Added below the order notes area.
$fields['order']['billing_newsletter'] = array(
'label' => 'Newsletter. Sent out 4-5 times a year.',
'type' => 'checkbox',
'required' => false,
'default' => 1, //This will pre-select the checkbox
'class' => array( 'form-row-wide' ),
'priority' => 26,
);
return $fields;
}
Result: adding custom fields to the checkout page.
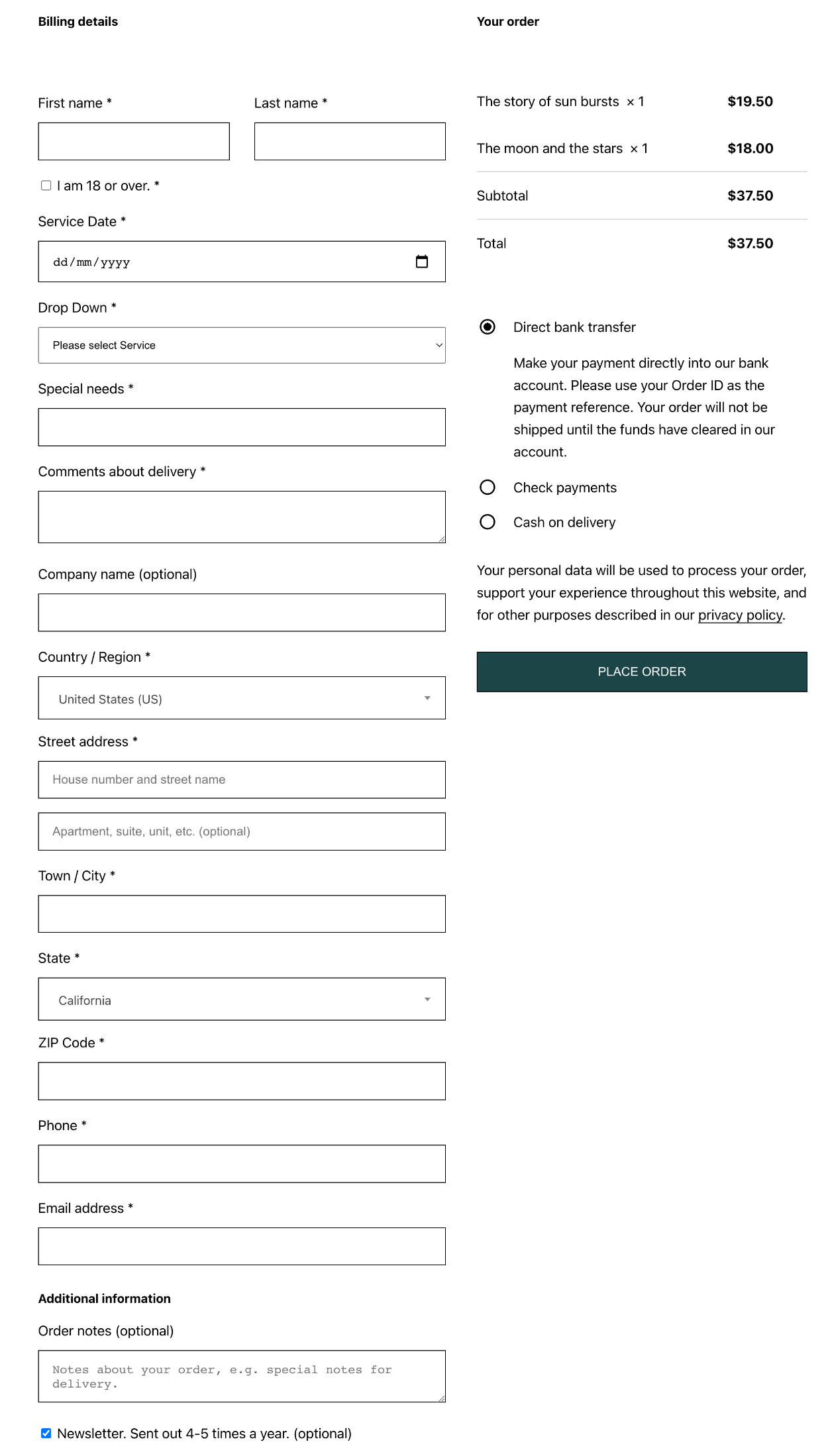
Save the custom checkout fields to the order meta.
First part is for the checkboxes. The next part is for adding the custom fields to the order edit page.
/* Custom fields that were added to the Order area will here be shown in the Billing area inside the WP backend WooCommerce -> Order and "Order Details" page. */
add_action('woocommerce_checkout_update_order_meta','my_custom_checkout_field_update_order_meta');
function my_custom_checkout_field_update_order_meta($order_id) {
if ( ! empty( $_POST['billing_age'] ) )
update_post_meta( $order_id, 'billing_age', $_POST['billing_age'] );
if ( ! empty( $_POST['billing_newsletter'] ) )
update_post_meta( $order_id, 'billing_newsletter', $_POST['billing_newsletter'] );
}
Display the custom field result on the order edit page (backend).
/* Display the custom field result on the order edit page (backend) when checkbox has been checked. https://stackoverflow.com/questions/45905237/add-a-custom-checkbox-in-woocommerce-checkout-which-value-shows-in-admin-edit-or
*/
add_action( 'woocommerce_admin_order_data_after_billing_address', 'display_custom_field_on_order_edit_pages', 10, 1 );
function display_custom_field_on_order_edit_pages( $order ){
$billing_age = get_post_meta( $order->get_id(), 'billing_age', true );
if( $billing_age == 1 )
echo '<p><strong>I am 18 or over: </strong> <span style="color:black;">Yes</span></p>';
$billing_newsletter = get_post_meta( $order->get_id(), 'billing_newsletter', true );
if( $billing_newsletter == 1 )
echo '<p><strong>Newsletter: </strong> <span style="color:black;">Yes</span></p>';
}
// Display fields in the backend Order details screen.
add_action( 'woocommerce_admin_order_data_after_billing_address', 'customfields_billing_checkbox_checkout_display' );
function customfields_billing_checkbox_checkout_display( $order ){
echo '<p><b>Text:</b> ' . get_post_meta( $order->get_id(), '_billing_text', true ) . '</p>';
echo '<p><b>Text Area:</b> ' . get_post_meta( $order->get_id(), '_billing_textarea', true ) . '</p>';
echo '<p><b>Date:</b> ' . get_post_meta( $order->get_id(), '_billing_date', true ) . '</p>';
echo '<p><b>Drop Down:</b> ' . get_post_meta( $order->get_id(), '_billing_dropdown', true ) . '</p>';
}
Result: backend Order details screen.
Backend: WooCommerce -> Orders -> Click to select an order.
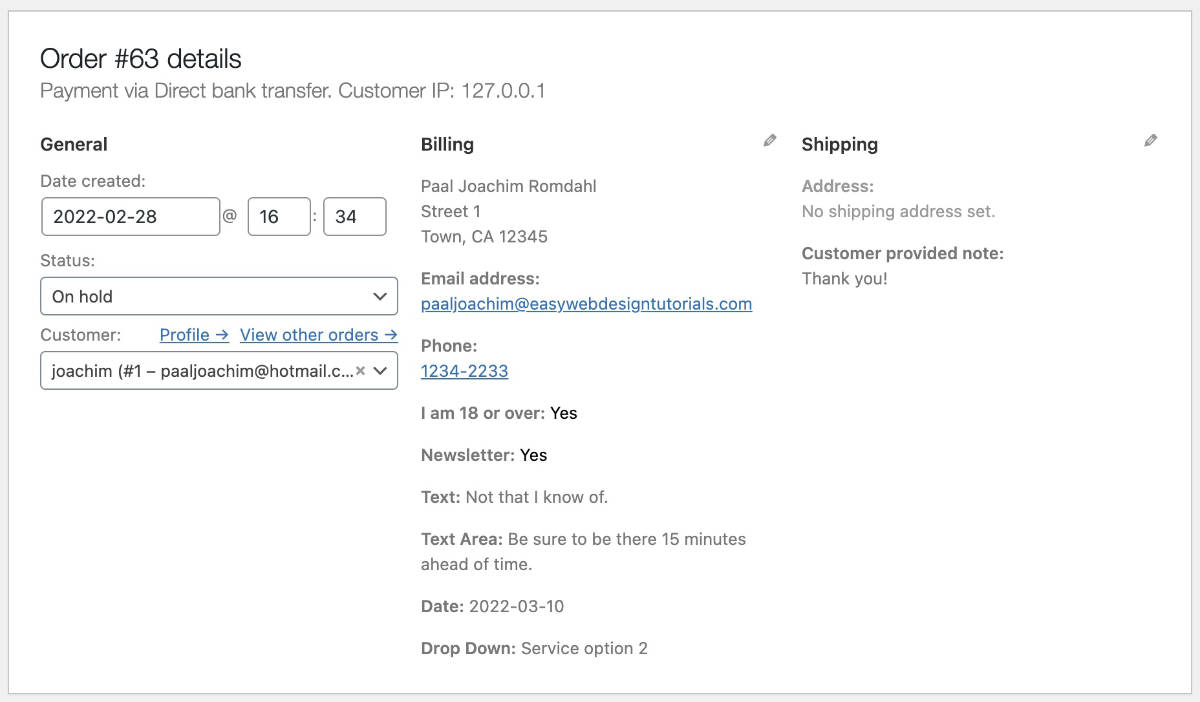
Notice how the custom fields are seen. “I am 18 or over” (checkbox), “Newsletter” (checkbox), “Text”, “Text Area”, “Date” and “Drop Down”. The names can be adjusted to better suit your needs.
Display fields in the customer e-mails.
/* Display fields in the admin and customer e-mails.
https://www.tychesoftwares.com/how-to-customize-woocommerce-order-emails/ */
add_action( 'woocommerce_email_after_order_table', 'ts_email_after_order_table', 10, 4 );
function ts_email_after_order_table( $order, $sent_to_admin, $plain_text, $email ) {
echo '<h2>Additional information</h2>';
echo '<p><strong>'.__('Text').':</strong> <br/>' . get_post_meta( $order->get_id(), '_billing_text', true ) . '</p>';
echo '<p><strong>'.__('Text Area').':</strong> <br/>' . get_post_meta( $order->get_id(), '_billing_textarea', true ) . '</p>';
echo '<p><strong>'.__('Date').':</strong> <br/>' . get_post_meta( $order->get_id(), '_billing_date', true ) . '</p>';
echo '<p><strong>'.__('Drop Down').':</strong> <br/>' . get_post_meta( $order->get_id(), '_billing_dropdown', true ) . '</p>';
$billing_age = get_post_meta( $order->get_id(), 'billing_age', true );
if( $billing_age == 1 )
echo '<p><strong>I am 18 or over: </strong> <span style="color:red; font-weight: bold;">Yes</span></p>';
$billing_newsletter = get_post_meta( $order->get_id(), 'billing_newsletter', true );
if( $billing_newsletter == 1 )
echo '<p><strong>Newsletter: </strong> <span style="color:black; font-weight: bold;">Yes</span></p>';
}
Result: Customer email.
The titles of each field can be named as you choose.
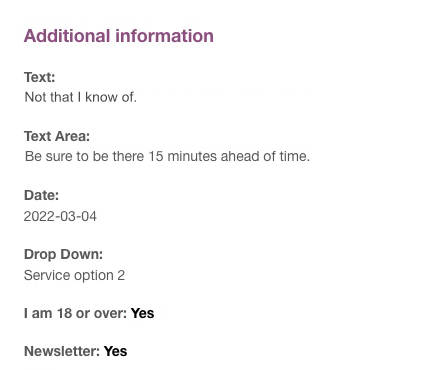
Resources:
https://businessbloomer.com/woocommerce-add-shipping-phone-checkout/
https://www.businessbloomer.com/woocommerce-add-custom-checkout-field-php/
https://www.businessbloomer.com/woocommerce-visual-hook-guide-checkout-page/
https://quadlayers.com/woocommerce-checkout-hooks/
https://quadlayers.com/customize-woocommerce-checkout-page/
( https://stackoverflow.com/questions/12958193/show-custom-field-on-order-in-woocommerce )
https://stackoverflow.com/questions/45905237/add-a-custom-checkbox-in-woocommerce-checkout-which-value-shows-in-admin-edit-or
https://www.tychesoftwares.com/how-to-customize-woocommerce-order-emails/
https://stackoverflow.com/questions/45905237/add-a-custom-checkbox-in-woocommerce-checkout-which-value-shows-in-admin-edit-or