How to modify the WooCommerce Cart page.
Here is the default cart page using the theme Twenty Twenty Two.
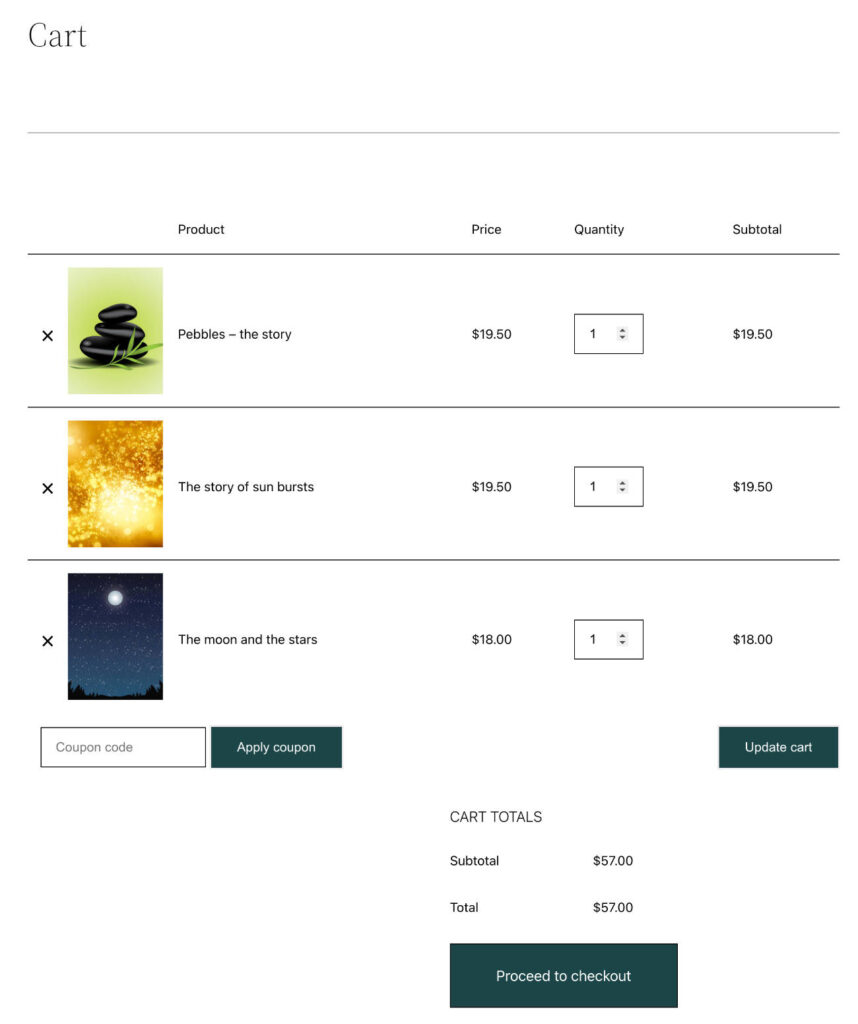
Add a continue button.
/* Add Continue Shopping Button on Cart Page & Checkout page:
https://gist.github.com/bradleysa/7d1448253097784daf94 */
add_action( 'woocommerce_before_cart_table', 'woo_add_continue_shopping_button_to_cart' );
add_action( 'woocommerce_before_checkout_form', 'woo_add_continue_shopping_button_to_cart' );
function woo_add_continue_shopping_button_to_cart() {
$shop_page_url = get_permalink( wc_get_page_id( 'shop' ) );
echo '<div class="woocommerce-message">';
echo ' <a href="'.$shop_page_url.'" class="button">Continue Shopping →</a> Do you need anything else?';
echo '</div>';
}
Result:
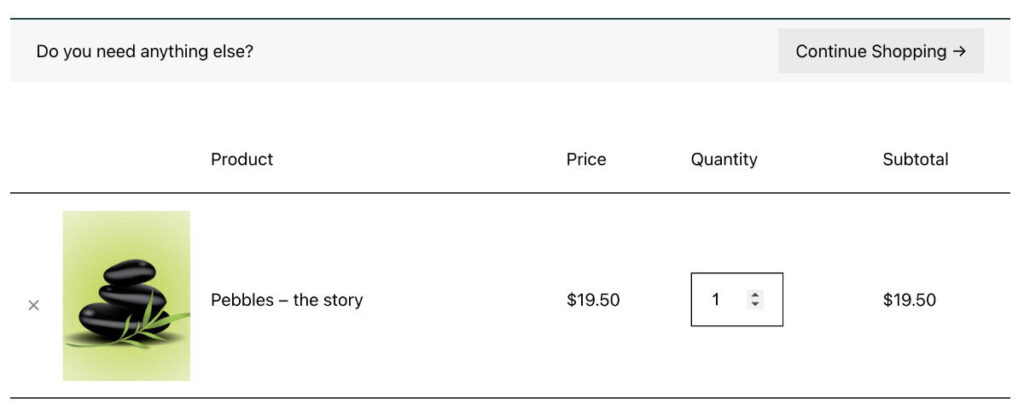
For a variation of the above code check my tutorial: Add a Continue Shopping button to Single product, Cart and Checkout pages.
Exchange x for a Fontawesome or Dashicon trash icon.
/* Exchange x with a Dashicon or Fontawesome trash icon.
https://woocommercecommunity.slack.com/archives/C1KAZ91E3/p1512580846000103?thread_ts=1512528458.000178&cid=C1KAZ91E3
WooCommerce: Change the “Remove this Item” Icon @ Cart
*/
// Load/Enqueue Fontawesome to get the Fontawesome icon to show up.
add_action( 'wp_enqueue_scripts', 'enqueue_font_awesome' );
function enqueue_font_awesome() {
wp_enqueue_style( 'font-awesome', '//maxcdn.bootstrapcdn.com/font-awesome/latest/css/font-awesome.min.css' );
}
// To use a Fontawesome icon add the following code.
function kia_cart_item_remove_link( $link, $cart_item_key ) {
return str_replace( '×', '<span class="cart-remove-icon"><i class="fa fa-trash" aria-hidden="true"></i></span>', $link );
}
add_filter( 'woocommerce_cart_item_remove_link', 'kia_cart_item_remove_link', 10, 2 );
// Load/Enqueue Dashicons to get the Dashicon icon to show up.
add_action( 'wp_enqueue_scripts', 'enqueue_dashicons' );
function enqueue_dashicons() {
wp_enqueue_style( 'dashicons' );
}
// To use a Dashicon add the following code.
function kia_cart_item_remove_link( $link, $cart_item_key ) {
return str_replace( '×', '<span class="cart-remove-icon"><span class="dashicons dashicons-trash"></span></span>', $link );
}
add_filter( 'woocommerce_cart_item_remove_link', 'kia_cart_item_remove_link', 10, 2 );
The CSS code:
.woocommerce a.remove,
.woocommerce a.remove:before {
/* font-family: 'dashicons'; */
/* content: "\f182"; */
font-size: 20px;
}
.woocommerce a.remove:hover {
color: red;
}
Result:
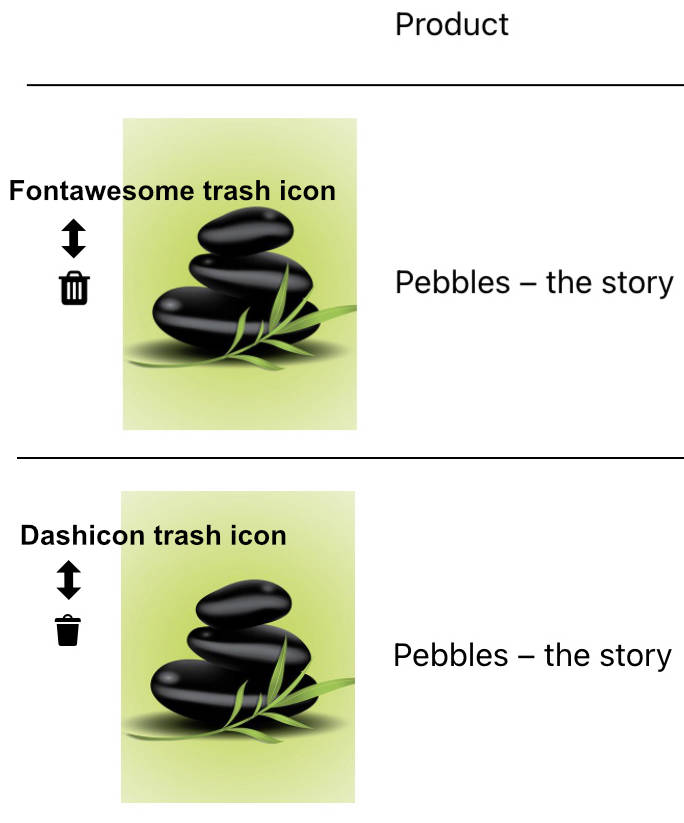
Only one individual product in cart.
Easily adjust through the below code the amount of product types to be added to the cart.
/* https://stackoverflow.com/questions/46007102/limit-the-number-of-cart-items-in-woocommerce/46008534#46008534
Checking and validating when products are added to cart */
add_filter( 'woocommerce_add_to_cart_validation', 'only_two_items_allowed_add_to_cart', 10, 3 );
function only_two_items_allowed_add_to_cart( $passed, $product_id, $quantity ) {
$cart_items_count = WC()->cart->get_cart_contents_count();
$total_count = $cart_items_count + $quantity;
// Change 1 to the number of product types you want to add to the cart.
if( $cart_items_count >= 1 || $total_count > 1 ){
// Set to false
$passed = false;
// Display a message
wc_add_notice( __( "You cannot have more than 1 type of product in the cart.", "woocommerce" ), "error" );
}
return $passed;
}
The result:
Showing what happens when trying to add a second product to the cart.
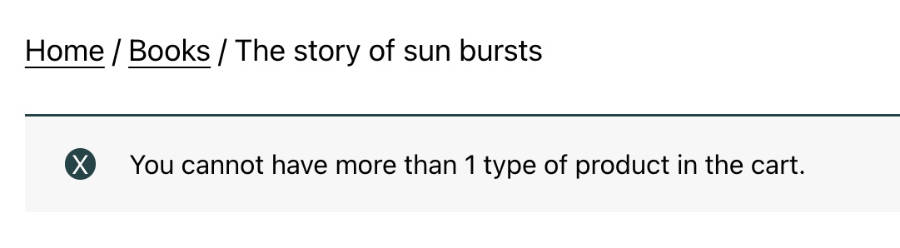
Display categories under product name.
/* Display categories under product name:
https://www.businessbloomer.com/woocommerce-display-categories-product-name-cart/ */
add_filter( 'woocommerce_cart_item_name', 'bbloomer_cart_item_category', 9999, 3 );
function bbloomer_cart_item_category( $name, $cart_item, $cart_item_key ) {
$product = $cart_item['data'];
if ( $product->is_type( 'variation' ) ) {
$product = wc_get_product( $product->get_parent_id() );
}
$cat_ids = $product->get_category_ids();
if ( $cat_ids ) $name .= '<br>' . wc_get_product_category_list( $product->get_id(), ', ', '<span class="posted_in">' . _n( 'Category:', 'Categories:', count( $cat_ids ), 'woocommerce' ) . ' ', '</span>' );
return $name;
}
The result:
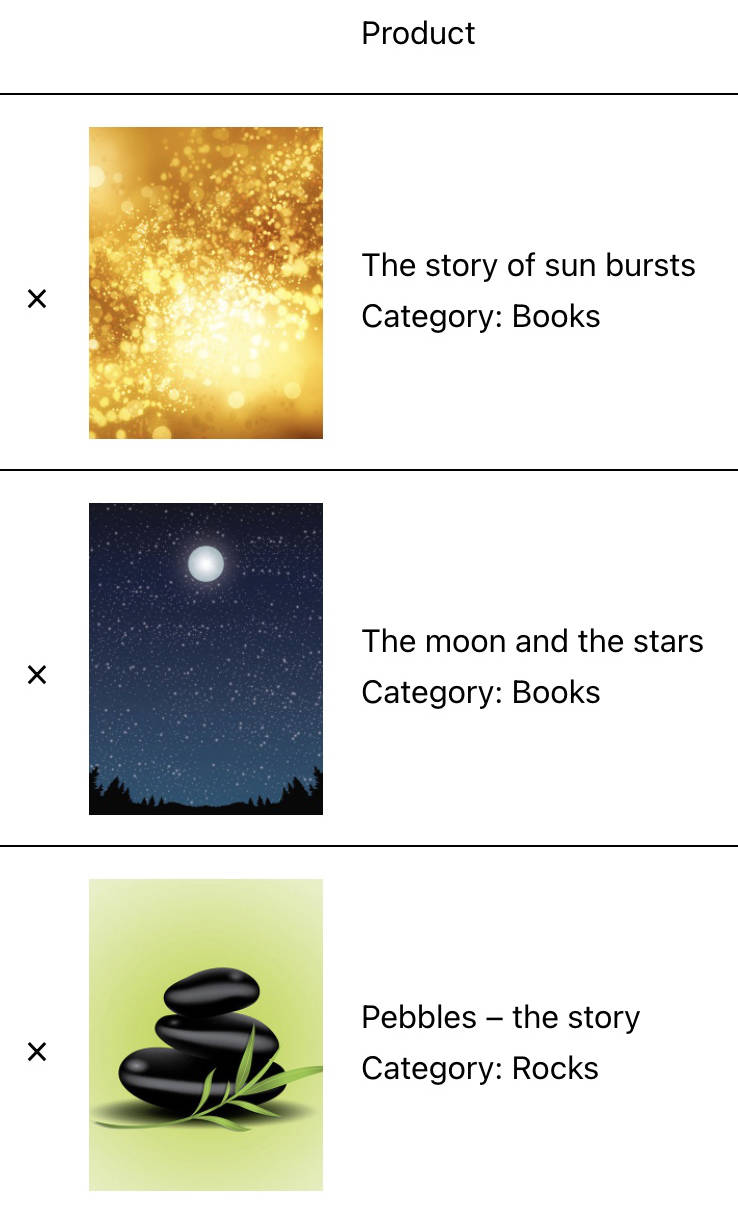
Resources:
https://businessbloomer.com/woocommerce-hide-price-add-cart-logged-users/
https://www.businessbloomer.com/woocommerce-visual-hook-guide-cart-page/
https://businessbloomer.com/woocommerce-change-remove-item-icon-cart