How to add and use JS scripts in WordPress.
A client who has the web site celias.no gave me the task of adding a calculator to her WordPress website. The calculator is used for non-profits organizations to calculate the profit when selling a certain amount of items.
Check the Calculator – JSFiddle to show the HTML, CSS and JS code in action.
Here is another JSFiddle calculator option.
The websites: godfortjeneste.no and dugnadsportalen.no/dugnadsguide have earlier used similar calculators.
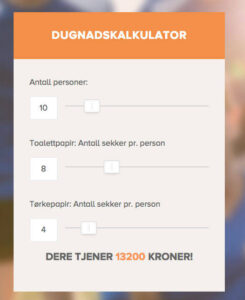
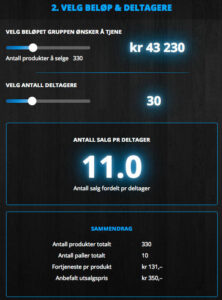
I asked for some help at the Advanced WordPress Facebook page and received a lot of help from Andrew and Jeppe! Awesome guys! Thanks for the help in getting this calculator working!!
By right clicking the calculator at godfortjeneste.no and selecting Inspect Element I found out that the site used nouislider to create the sliders seen in the form.
The code I used for my client celias.no for creating the profit calculator.
functions.php
/*---------- Dugnadskalkulator - Profit Calculator - functions.php file --------*/
function dugnad_js_css(){
wp_register_style( 'dugnadskalkulator', get_template_directory_uri() . '/assets/css/dugnadskalkulator.css');
wp_enqueue_style( 'dugnadskalkulator' );
wp_register_script( 'dugnadskalkulator', get_stylesheet_directory_uri() . '/assets/js/dugnadskalkulator.js', array('jquery'));
wp_enqueue_script( 'dugnadskalkulator' );
}
add_action('wp_enqueue_scripts', 'dugnad_js_css');
wp_enqueue
wp_enqueue_script( $handle, $source, $dependencies, $version, $in_footer );
wp_enqueue_style( $handle, $source, $dependencies, $version, $media );
$handle: unique name for script or style.
Example:’colorbox‘
$source: the url of the script/style.
Example: get_template_directory_uri() . ‘/colorbox/jquery.colorbox-min.js
$dependencies: An array of assets the script/style depends on. Loaded before the enqueued script.
Example: array(‘jquery’)
$version: A version number which will be appended to the query. To make sure that the user receives the correct version.
Example: ‘1.0.0’
$in_footer: For loading scripts in the wp_footer() at the bottom of the page.
Example: true
$media: How the media should be displayed.
Example: screen, print. handheld etc.
dugnadskalkulator.js
Jeppe created a codepen dugnadskalkulator code based on nouislider containing the code for the HTML, CSS and JS files. I copied and modified the Codepen JS code and added it to a new JS file I named dugnadskalkulator.js and placed the file into the assets/js folder.
function updateRanges(){
// update all ranges' outputs (visual effect only
// since we do math on range values and not outputs)
jQuery('input[type="range"]').each(function(){
var rangeNum = jQuery(this).val(),
rangeFor = jQuery(this).attr('name');
// Old code
//jQuery('output[for="'+rangeFor+'"]').val(rangeNum);
// New code that also makes the calculator work in IE
jQuery('output[for="'+rangeFor+'"]').replaceWith('<output for ="'+rangeFor+'">'+rangeNum+'</output>');
});
// update the total value
var rangeVals = {
'one' : parseInt(jQuery('input[name="r10"]').val()), // Hvor mye penger ønsker gruppen å tjene? (How much money does the group want to earn?)
'two' : parseInt(jQuery('input[name="r20"]').val()), // Velg antall deltagere i gruppen:
(Select amount of participants in the group:)
};
// Do the math
jQuery('.resultat-salgprodukter span').html(rangeVals.one / 70); // Antall produkter å selge = (Amount of products to sell)
jQuery('.resultat-salgdeltager span').html(Math.round((rangeVals.one / 70 / rangeVals.two) * 1) / 1); // Antall salg pr. deltager (amount of sales per participant): 1 = no decimals, 100 = 2 decimals */
// Sammendrag
jQuery('.resultat-produktertotalt span').html(Math.round((rangeVals.one / 70) * 1) / 1); // Antall Produkter Totalt: (Total product amount)
jQuery('.resultat-kartonger span').html(rangeVals.one / 70 / 20); // Antall Kartonger a 20 esker
(Amount of cartons of 20 boxes)
}
// Code that helps the calculator work in IE
jQuery('input').change( function() {
updateRanges()
});
To limit decimals one way is to use the (Math.round((rangeVals.one / 70 / rangeVals.two) * 1) / 1); calculation. 1 = no decimals. 100 = 2 decimals.
Here is additional JS if the code is to be used in a Method 1: In a plugin and
Method 2:In a theme. Thanks to Jason for the following code:
(Just remember to also add the CSS similar to what I did with the above code I added to the functions.php file.)
dugnadskalkulator.css
I added the following CSS to a new filed I named dugnadskalkulator.css. Which I placed into the assets/css folder. The code I decided to use is the full CSS for the styled range inputs from the CSS Styling Range Inputs article from CSS Tricks.
/* the full form area */
#calc-form {
border: 1px solid #333;
/* padding: 17px; */
border-radius: 7px;
box-shadow: 0px 3px 5px #444;
width: 300px;
}
#calc-heading {
background: #a0ce4e;
color: #fff;
font-size: 26px;
border-bottom: 1px solid #333;
padding: 17px;
border-radius: 7px 7px 0px 0px;
font-family: times, serif;
text-align: center;
text-shadow: 1px 1px 1px #0a0909;
letter-spacing: 1.8px;
}
#calc-content {
padding: 13px;
font-size: 18px;
background: #f8f1f1;
}
.styled-output {
clear: both;
/* text-align: center; */
margin: 5px 0 0 0;
padding: 5px 10px 0 9px;
/* width: 75px; */
text-align: center;
border: 1px solid #dbc9c9;
color: #67990f;
font-size: 20px;
}
.resultat-salgdeltager {
text-align: center;
color: #67990f;
margin-bottom: 7px;
font-size: 18px;
}
#calc-sammendrag-heading {
font-size: 26px;
border-bottom: 1px solid #333;
padding: 17px;
border-radius: 7px 7px 0px 0px;
font-family: times, serif;
text-align: center;
}
.calc-sammendrag {
text-align: left;
/* border: 1px solid #333; */
padding: 4px;
}
input[type=range] {
-webkit-appearance: none;
margin: 5px 0 25px 0;
width: 100%;
}
input[type=range]:focus {
outline: none;
}
input[type=range]::-webkit-slider-runnable-track {
width: 100%;
height: 8.4px;
cursor: pointer;
animate: 0.2s;
box-shadow: 1px 1px 1px #000000, 0px 0px 1px #0d0d0d;
background: #3071a9;
border-radius: 1.3px;
border: 0.2px solid #010101;
}
input[type=range]::-webkit-slider-thumb {
box-shadow: 1px 1px 1px #000000, 0px 0px 1px #0d0d0d;
border: 1px solid #000000;
height: 22px;
width: 25px; /* Changed */
border-radius: 10px; /* 3px */
background: #a0ce4e; /* #fff */
cursor: pointer;
-webkit-appearance: none;
margin-top: -7px; /* Changed */
}
input[type=range]:focus::-webkit-slider-runnable-track {
background: #367ebd;
}
input[type=range]::-moz-range-track {
width: 100%;
height: 8.4px;
cursor: pointer;
animate: 0.2s;
box-shadow: 1px 1px 1px #000000, 0px 0px 1px #0d0d0d;
background: #3071a9;
border-radius: 1.3px;
border: 0.2px solid #010101;
}
input[type=range]::-moz-range-thumb {
box-shadow: 1px 1px 1px #000000, 0px 0px 1px #0d0d0d;
border: 1px solid #000000;
height: 36px;
width: 16px;
border-radius: 3px;
background: #ffffff;
cursor: pointer;
}
input[type=range]::-ms-track {
width: 100%;
height: 8.4px;
cursor: pointer;
animate: 0.2s;
background: transparent;
border-color: transparent;
border-width: 16px 0;
color: transparent;
}
input[type=range]::-ms-fill-lower {
background: #2a6495;
border: 0.2px solid #010101;
border-radius: 2.6px;
box-shadow: 1px 1px 1px #000000, 0px 0px 1px #0d0d0d;
}
input[type=range]::-ms-fill-upper {
background: #3071a9;
border: 0.2px solid #010101;
border-radius: 2.6px;
box-shadow: 1px 1px 1px #000000, 0px 0px 1px #0d0d0d;
}
input[type=range]::-ms-thumb {
box-shadow: 1px 1px 1px #000000, 0px 0px 1px #0d0d0d;
border: 1px solid #000000;
height: 36px;
width: 16px;
border-radius: 3px;
background: #ffffff;
cursor: pointer;
}
input[type=range]:focus::-ms-fill-lower {
background: #3071a9;
}
input[type=range]:focus::-ms-fill-upper {
background: #367ebd;
}
Style.css
To get the CSS inside dugnadskalkulator.css working one has to also use the import code in the child theme stylesheet. I added the following code close to the top of the style.css:
/* Import CSS files */
@import url(assets/css/dugnadskalkulator.css);
HTML
I modified the HTML Jeppe wrote in the codepen dugnadskalkulator.
<div id="calc-form">
<!-- <form oninput="updateRanges()"> -->
<form oninput="updateRanges()" onchange="updateRanges()"> <!-- For IE--->
<div id="calc-heading">Dugnadskalkulator</div>
<div id="calc-content">
<div class="slider">
<div class="styled-output">
<output for="r1">1</output></div> Antall personer / percipients
<input type="range" name="r1" step="1" min="1" max="40" value="1">
</div>
<div class="slider">
<div class="styled-output">
<output for="r2">1</output></div> Salg / Sale
<input type="range" name="r2" step="1" min="0" max="40" value="1">
</div>
<!-- Information about r3 third slider has been removed since I believe I only need two sliders. If it is needed I would just copy r2 and adjust.--->
<div class="result">
Du tjener / You earn <span>220</span> Kr
</div>
</div>
</form>
</div>
The HTML for the more complex Profit Calculator:
<div id="calc-form">
<form oninput="updateRanges()">
<div id="calc-heading">Dugnadskalkulator</div>
<div id="calc-content">
<div class="slider">Hvor mye penger ønsker gruppen å tjene?
<div class="styled-output"><output for="r10">1400</output>kr</div>
<input type="range" name="r10" step="1400" min="1400" max="160000" value="0">
</div>
<p> <div class="resultat-salgprodukter">Antall produkter å selge: <span>20</span> <br /></p>
<div class="slider">Velg antall deltagere i gruppen: </br>
<div class="styled-output"><output for="r20">1</output></div>
<input type="range" name="r20" step="1" min="1" max="40" value="1">
</div>
</div>
<div class="resultat-salgdeltager">Antall bursdagsesker pr. deltager: <br/><span>20</span></div>
<div id="calc-sammendrag-heading">Sammendrag</div>
<div class="calc-sammendrag">
<p>
<div class="resultat-salgprodukter">
Antall Produkter Totalt: <span>20</span>
</div>
<div class="resultat-kartonger">
Antall Kartonger a 20 Esker: <span>1</span>
</div>
Fortjeneste pr. Produkt 70 Kr
</br>Anbefalt utsalgspris 130kr
</div>
</div>
</form>
</div>
NB! The code in this tutorial does not work in Internet Explorer.
dugnad-calc.php
Adding the HTML into the PHP plugin file. Named the file dugnad-calc.php, and added the file into a folder I named dugnad-calc.
Here is the code:
<?php
/*
Plugin name: Dugnadscalculator Shortcode
Description: Makes a shortcode [dugnadskalkulator] to place a dugnadscalculator anywhere on your page.
Author: Paal Joachim Romdahl
Author uri: http://www.easywebdesigntutorials.com/
*/
/*
------
Register the dugnad script and style, but do not enqueue */
add_action('wp_enqueue_scripts','dugnad_script_reg');
function dugnad_script_reg(){
wp_register_script(
'dugnadskalkulator',
plugins_url('dugnad-calc').'/assets/script.js',
array('jquery'),
null,
true
);
wp_register_style(
'dugnadskalkulator',
plugins_url('dugnad-calc').'/assets/style.css',
null,
null,
'all'
);
}
/*
------
Register the dugnad shortcode - [dugnadskalkulator] */
add_shortcode( 'dugnadskalkulator', 'dugnad_shortcode' );
function dugnad_shortcode() {
// Enqueue our registered script to be added in the footer, when our shortcode is used.
// Should work, according to http://mikejolley.com/2013/12/sensible-script-enqueuing-shortcodes/
wp_enqueue_script('dugnadskalkulator');
wp_enqueue_style('dugnadskalkulator');
ob_start(); // Begin output buffer
?>
<div id="calc-form">
<form oninput="updateRanges()" onchange="updateRanges()"> <!-- For IE--->
<div id="calc-heading">Dugnadskalkulator</div>
<div id="calc-content">
<div class="calcslider">Hvor mye penger ønsker gruppen å tjene?
<div class="styled-output"><output for="r10">1600</output>kr</div>
<input type="range" name="r10" step="1600" min="1600" max="160000" value="0">
</div>
<div class="resultat-salgprodukter">Antall produkter å selge: <span>20</span> <br /></div>
<div class="calcslider">Velg antall deltagere i gruppen: <br /></div>
<div class="styled-output"><output for="r20">1</output></div>
<input type="range" name="r20" step="1" min="1" max="40" value="1">
<div class="resultat-salgdeltager">Antall produkter pr. deltager: <br><span>20</span></div>
<div id="calc-sammendrag-heading">Sammendrag</div>
<div class="calc-sammendrag">
<div class="resultat-salgprodukter">
Antall produkter totalt: <span>20</span>
</div>
<!-- <div class="resultat-kartonger">
Antall kartonger a 20 esker: <span>1</span>
</div> -->
Fortjeneste pr. produkt: 80 Kr<br />
Anbefalt utsalgspris: <b>150kr</b>
</div>
</div>
</form>
</div>
<?php
return ob_get_clean(); // return and clean output buffer
}
I made the calculator into a plugin with Jeppes help. It is located here:
https://github.com/paaljoachim/calculator
Overview:
dugnad-calc folder
-dugnad-calc.php
–assets folder
— script.js (dugnadskalkulator.js) script.js
— style.css (dugnadskalkulator.css) style.css
The result:
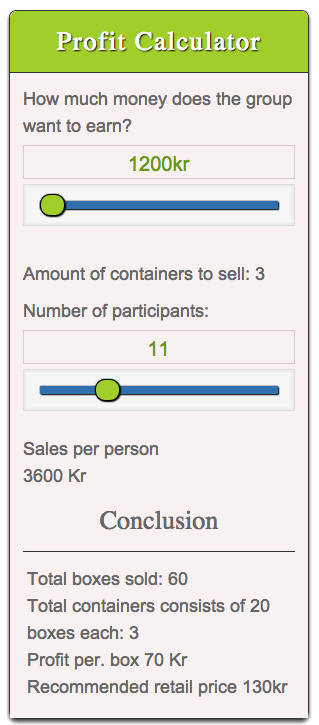
Resources:
https://ithemes.com/2015/02/17/adding-scripts-wordpress-right-way/
https://community.getbeans.io/discussion/how-paste-a-code-in-the-head-of-the-site/
https://css-tricks.com/styling-cross-browser-compatible-range-inputs-css/
https://codepen.io/Sneppe/pen/jPNWqv
https://refreshless.com/nouislider/